
Download chrome for macos catalina. Dropbox for mac app. Regular Expression Character Classes ab-d One character of: a, b, c, d ^ab-d One character except: a, b, c, d b Backspace character d: One digit D: One non-digit s: One whitespace S: One non-whitespace w: One word character W: One non-word character. Mac os big sur for virtualbox.
Special characters
- Python unittest Assertions Enjoy this cheat sheet at its fullest within Dash, the macOS documentation browser.
- Pythex is a quick way to test your Python regular expressions. Try writing one or test the example. Match result: Match captures: Regular expression cheatsheet Special characters escape special characters. Matches any character ^ matches beginning of string $ matches end of string 5b-d.
.Default: Match any character except newline.DOTALL: Match any character including newline^Default: Match the start of a string^MULTILINE: Match immediatly after each newline$Match the end of a string$MULTILINE: Also match before a newline*Match 0 or more repetitions of RE+Match 1 or more repetitions of RE?Match 0 or 1 repetitions of RE*?, *+, ??Match non-greedy as
few characters as possible{m}Match exactly
m copies of the previous RE{m,n}Match from
m to
n repetitions of RE{m,n}?Match non-greedyEscape special characters[]Match a
set of characters|
RE1|
RE2: Match either RE1
or RE2 non-greedy(..)Match RE inside parantheses and indicate start and end of a groupWith RE is the resulting regular expression.
Special characters must be escaped with if it should match the character literally
Methods of 're' module
re.
compile(
pattern,
flags=0)Compile a regular expression pattern into a regular expression object. Can be used with
match(),
search() and othersre.
search(
pattern,
string,
flags=0Search through
string matching the first location of the RE. Returns a
match object or
Nonere.
match(
pattern,
string,
flags=0)If zero or more characters at the beginning of a string match
pattern return a
match object or
Nonere.
fullmatch(
pattern,
string,
flags=0)If the whole
string matches the
pattern return a
match object or
Nonere.
split(
pattern,
string,
maxsplit=0,
flags=0)Split
string by the occurrences of
patternmaxsplit times if non-zero. Returns a
list of all groups.re.
findall(
pattern,
string,
flags=0)Return all non-overlapping matches of
pattern in
string as
list of strings.re.
finditer(
pattern,
string,
flags=0)Return an
iterator yielding
match objects over all non-overlapping matches for the
pattern in
stringre.
sub(
pattern,
repl,
string,
count=0,
flags=0)Return the
string obtained by replacing the leftmost non-overlapping occurrences of
pattern in
string by the
replacementrepl.
repl can be a function.re.
subn(
pattern,
repl,
string,
count=0,
flags=0)Like
sub but return a tuple (
new_string,
number_of_subs_made)re.
escape(
pattern)Escape special characters in
patternre.
purge()Clear the regular expression cache
Raw String Notation
In raw string notation
r'text'
there is no need to escape the backslash character again.
>>> re.match(r'W(.)1W', ' ff ')
<re.Match object; span=(0, 4), match=' ff '>
>>> re.match('W(.)1W', ' ff ')
<re.Match object; span=(0, 4), match=' ff '>
Reference
https://docs.python.org/3/howto/regex.htmlhttps://docs.python.org/3/library/re.html
Extensions
(?..)This is the start of an extension(?aiLmsux)The letters set the correspondig flags
See flags(?:..)A non-capturing version of regular parantheses(?P<name>..)Like regular paranthes but with a
named group(?P=name)A backreference to a
named group(?#..)A comment(?=..)
lookahead assertion: Matches if
.. matches next without consuming the string(?!..)
negative lookahead assertion: Matches if
.. doesn't match next(?<=..)
positive lookbehind assertion: Match if the current position in the string is preceded by a match for
.. that ends the current position(?<!..)
negative lookbehind assertion: Match if the current position in the string is
not preceded by a match for
..(?(id/name)yes-pattern|no-pattern)Match with
yes-pattern if the group with gived
id or
name exists and with
no-pattern if not
Match objects
Match.
expand(
template)Return the string obtained by doing backslash substitution on
template, as done by the
sub() methodMatch.
group(
[group1,..
])
Regex Python Cheat Sheet Pdf
Returns one or more subgroups of the match. 1 Argument returns
string and more arguments return a
tuple.Match.
__getitem__(
g)Access groups with m[0], m[1] ..Match.
groups(
default=None)Return a
tuple containing all the subgroups of the matchMatch.
groupdict(
default=None)Return a
dictionary containing all the
named subgroups of the match, keyed by the subgroup name.Match.
start(
[group]Match.
end(
[group])Return the indices of the start and end of the substring matched by
groupMatch.
span(
[group])For a match
m, return the 2-tuple
(m.start(group) m.end(group))
Match.
posThe value of
pos which was passed to the
search() or
match() method of the
regex objectMatch.
endposLikewise but the value of
endposMatch.
lastindexThe integer index of the last matched capturing group, or
None
.Match.
lastgroupThe name of the last matched capturing group or
None
Match.
reThe
regular expression object whose
match() or
search() method produced this match instanceMatch.
stringThe string passed to
match() or
search()Special escape characters
AMatch only at the start of the stringbMatch the empty string at the beginning or end of a wordBMatch the empty string when
not at the beginning or end of a worddMatch any
Unicode decimal digit this includes [0-9]DMatch any character which is
not a decimal digitsMatch
Unicode white space characters which includes [ tnrfv]SMatches any character which is
not a whitespace character. The opposite of swMatch
Unicode word characters including [a-zA-Z0-9_]W
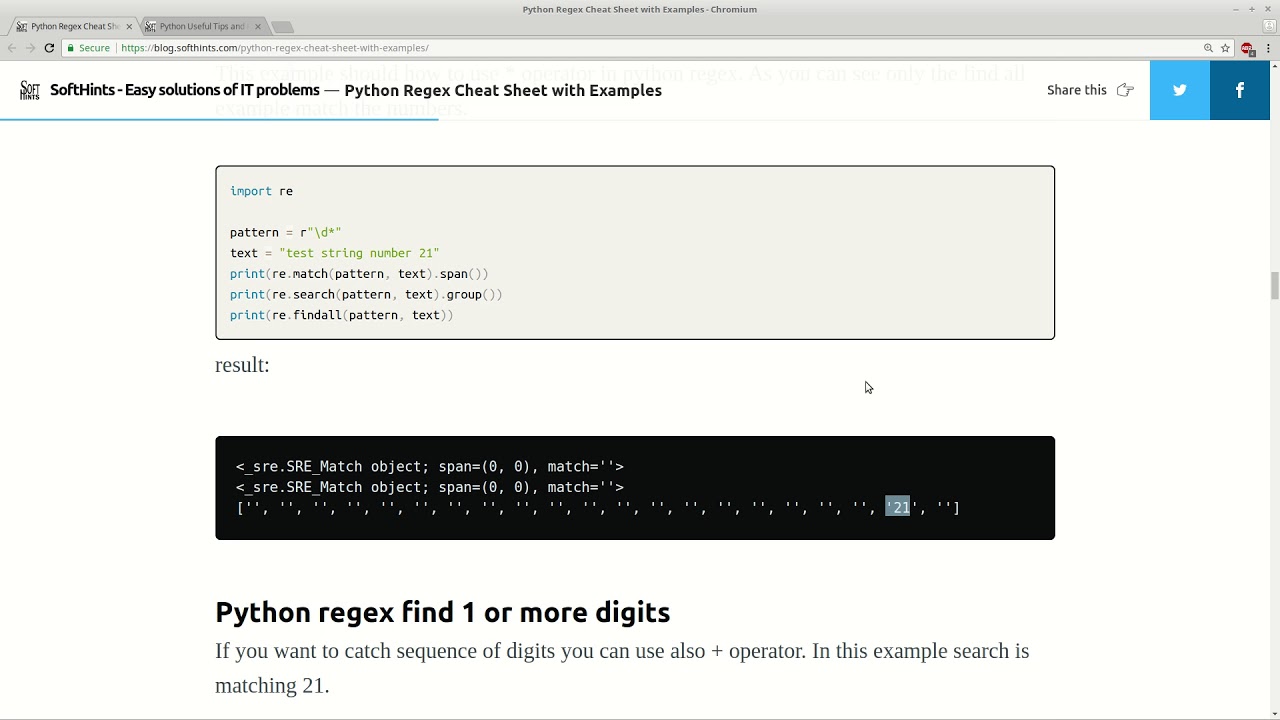
Match the opposite of wZMatch only at the end of a string
Regular Expression Objects
Pattern.
search(
string[,
pos[,
endpos]])See
re.search()
.
pos gives an index where to start the search.
endpos limits how far the string will be searched.Pattern.
match(
string[,
pos[,
endpos]])Likewise but see
re.match()
Pattern.
fullmatch(
string[,
pos[,
endpos]])Likewise but see
re.fullmatch()
Pattern.
split(
string,
maxsplit=0)Identical to
re.split()
Pattern.
findall(
Re Cheat Sheet
string[,
pos[,
endpos]])Similar to
re.findall()
but with additional parameters
pos and
endposPattern.
finditer(
string[,
pos[Regular Expression Tester
,
endpos]])Similar to
re.finditer()
but with additional parameters
pos and
endposRegular Expression Match Exact String
Pattern.
sub(
repl,
string,
count=0)Identical to
re.sub()
Pattern.
subn(
repl,
string,
count=0)Identical to
re.subn()
Pattern.
flagsThe regex matching flags.Pattern.
groupsThe number of capturing groups in the patternPattern.
groupindexA dictionary mapping any symbolic group names to group membersPattern.
patternThe pattern string from which the pattern object was compiledThese objects are returned by the
re.compile()
method
Flags
ASCII, AASCII-only matching in w, b, s and dIGNORECASE, Iignore caseLOCALE, Ldo a local-aware matchMULTILINE, Mmultiline matching, affecting
^
and
$
DOTALL, Sdot matches alluunicode matching (just in (?aiLmsux))VERBOSE, XverboseFlags are used in (?aiLmsux-imsx:..) or (?aiLmsux) or can be accessed with re.
FLAG. In the first form flags are set or removed.
This is useful if you wish to include the flags as part of the regular expression, instead of passing a flag argument to the re.compile() function
Regular Expression Cheat Sheet
developmentpythonregexregularprogrammingexpression